Embedded Programming
Overview of week 4
Group Assignment
Demonstrate and compare the toolchains and development workflows for available embedded architectures.
Individual Assignments
Browse through the data sheet for your microcontroller write a program for a microcontroller, and simulate its operation, to interact (with local input &/or output devices) and communicate (with remote wired or wireless connections).
Individual Assignment :
Arduino
Arduino is an open-source electronics platform with hardware and software designed for ease of use. It features microcontroller-based boards like Arduino Uno (ATmega328P) and supports C/C++ programming. The Arduino IDE simplifies coding and uploading programs. It interfaces with sensors, LEDs, motors, and other components. Its open-source nature allows customization and improvements. With extensive community support, it is widely used in education, prototyping, and IoT projects.
In my exploration, I am using the Arduino Uno, which has allowed me to learn basic simulations in software platforms.
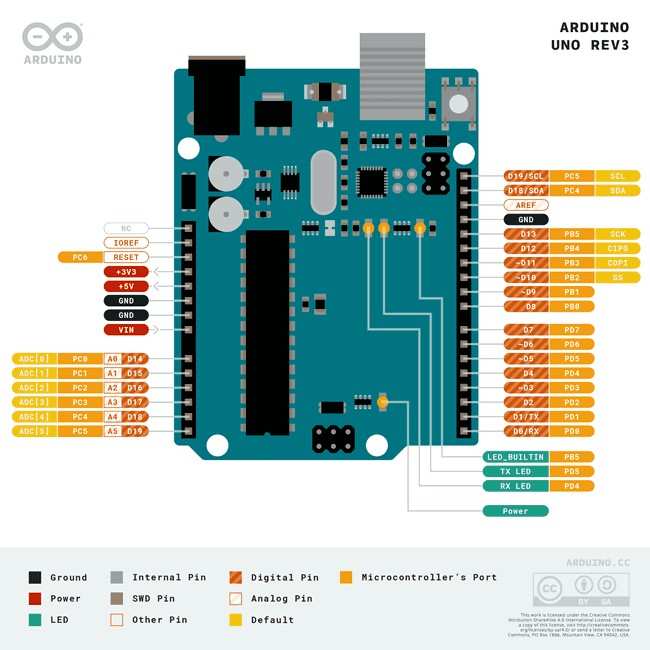
ATmega328P
The ATmega328P is an 8-bit AVR microcontroller developed by Microchip Technology. It is widely used in embedded systems.
Specification
- Architecture: 8-bit AVR RISC
- Clock Speed: Up to 20 MHz (16 MHz in Arduino Uno)
- Flash Memory: 32 KB (for storing programs)
- EEPROM: 1 KB (for non-volatile data storage)
- GPIO Pins: 23 I/O pins (including 6 PWM and 6 ADC channels)
- Communication Interfaces: UART, SPI, I2C
Datasheet
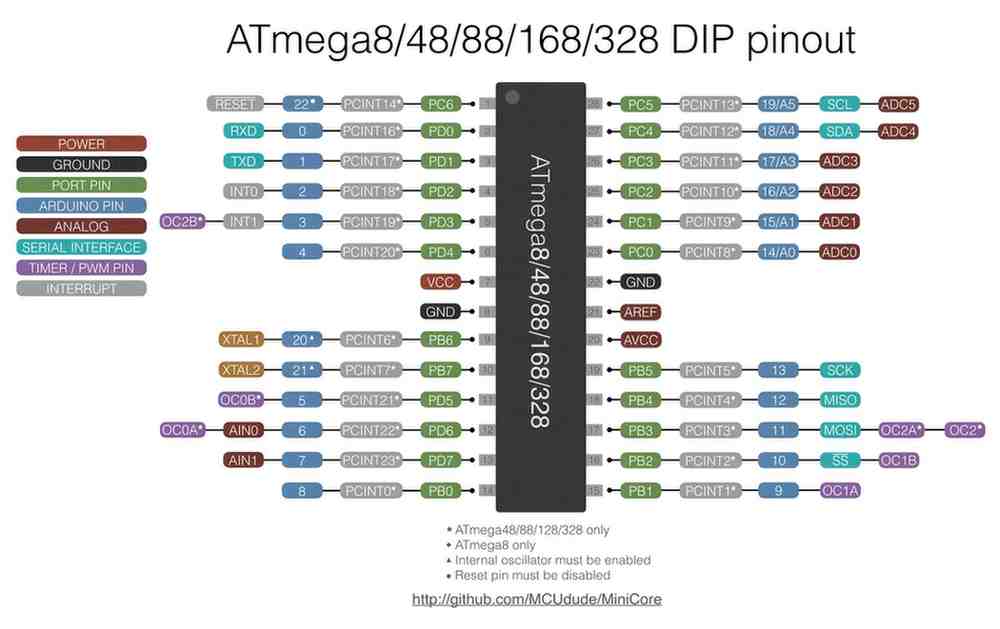
Tinkercad
Tinkercad is a free, web-based platform by Autodesk for 3D design, circuit simulation, and Arduino coding. It allows users to create 3D models, simulate electronic circuits, and program Arduino using block or text-based coding. With a user-friendly interface, it is ideal for beginners in electronics, robotics, and design. No installation is needed, as it runs directly in a web browser.
I used Tinkercad for basic Arduino simulations. I explored the interface of tinkercad and got some ideas how to do the Components arrangements, connections btw the others, coding and simulations.
LED blink
First i did the basic simulation of LED blink, I take the arduino in the library and also collect the LED and resistor, Connect them as per the code which is I given in the Coding interface.
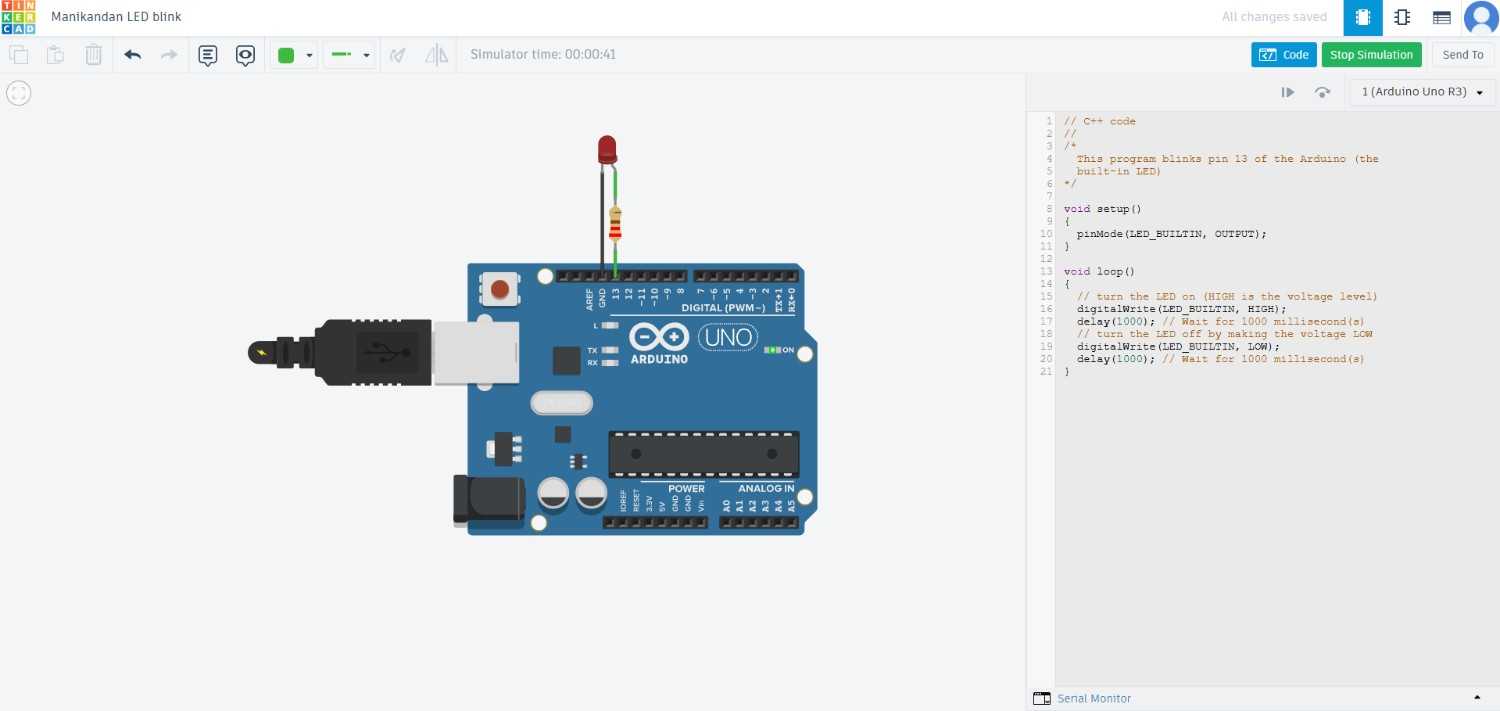
void setup()
{
pinMode(LED_BUILTIN, OUTPUT);
}
void loop()
{
digitalWrite(LED_BUILTIN, HIGH);
delay(1000);
digitalWrite(LED_BUILTIN, LOW);
delay(1000);
}
LCD Display
After that I do the simulations with utilize the LCD display,
Adafruit_LiquidCrystal lcd(0);
void setup()
{
lcd.begin(16, 2);
lcd.setCursor(1,0);
lcd.print("Fablab Trichy");
}
void loop()
{
lcd.setBacklight(1);
delay(100);
lcd.setBacklight(0);
delay(10);
}
Servo mechanism
Further I did the Servo actuation by controlling the code as well
#include <Servo.h>
int pos = 0;
Servo servo_10;
void setup()
{
servo_10.attach(2, 500, 2500);
}
void loop()
{
for (pos = 0; pos <= 90; pos += 1) {
servo_10.write(pos);
delay(1);
}
}
ESP
ESP (Espressif Systems) is a series of low-power microcontrollers widely used in IoT applications. It offers built-in Wi-Fi and Bluetooth connectivity, making it ideal for wireless communication. ESP modules support multiple programming environments, including Arduino, MicroPython, and ESP-IDF. They are known for their high performance, low power consumption, and cost-effectiveness. ESP microcontrollers integrate various peripherals such as ADC, DAC, PWM, and GPIOs. They are commonly used in smart home devices, automation, and embedded systems. The most popular models include ESP8266 and ESP32, with ESP32 offering enhanced features like dual-core processing.
I explored ESP32 microcontrollers and gained knowledge that may make it a suitable choice for my final project.
ESP32
The ESP32 is a powerful Wi-Fi and Bluetooth-enabled microcontroller developed by Espressif Systems. It features a dual-core 32-bit Xtensa LX6 processor, running up to 240 MHz, with 520 KB SRAM and 4 MB flash memory (varies by model). It supports Wi-Fi (802.11 b/g/n), Bluetooth 4.2/BLE, GPIOs, ADC, DAC, SPI, I2C, UART, and PWM. Known for its low power consumption and high performance, it is widely used in IoT, robotics, automation, and embedded systems.
Specification
- Architecture: 32-bit Xtensa LX6 (dual-core)
- Clock Speed: Up to 240 MHz
- Flash Memory: Up to 16 MB (varies by model)
- SRAM: 520 KB (internal)
- GPIO Pins: Up to 34 I/O pins
- ADC: 18 channels (12-bit resolution)
- DAC: 2 channels (8-bit resolution)
- Communication Interfaces: UART, SPI, I2C, I2S, CAN, PWM
- Wireless Connectivity: Wi-Fi (802.11 b/g/n), Bluetooth 4.2 + BLE
- Operating Voltage: 3.3V
Datasheet
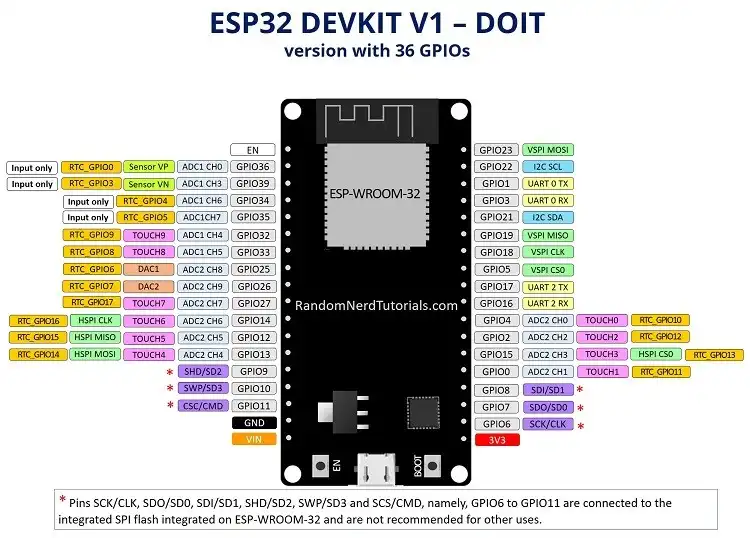
Seeed studio
Seeed Studio is a hardware innovation platform that provides open-source electronic modules, development boards, and IoT solutions. It offers products like Seeeduino (Arduino-compatible boards), Grove sensors, XIAO microcontrollers, and Edge AI solutions. Known for its rapid prototyping, industrial IoT, and AI hardware, Seeed Studio supports makers, students, and engineers in building embedded systems and smart devices.
Furthermore, I explored this in greater depth and practiced working with it.
XIAO-ESP32-C3
The Seeed Studio XIAO ESP32-C3 is a compact Wi-Fi + Bluetooth 5 (BLE) microcontroller based on the ESP32-C3 (RISC-V single-core CPU @160 MHz). It features 4 MB Flash, 400 KB SRAM, and supports GPIO, ADC, PWM, UART, SPI, and I2C. With its small size (21×17.5 mm), low power consumption, and Type-C USB, it is ideal for IoT, wearables, and edge computing applications.
Specification
- Architecture: 32-bit RISC-V (single-core)
- Architecture: 32-bit RISC-V (single-core)
- Clock Speed: Up to 160 MHz
- Flash Memory: 4 MB
- SRAM: 400 KB
- GPIO Pins: 11 I/O pins (supports ADC, PWM, I2C, SPI, UART)
- Wireless Connectivity: Wi-Fi (802.11 b/g/n, 2.4 GHz), Bluetooth 5 (BLE)
- USB Interface: USB Type-C (native)
- Operating Voltage: 3.3V
- Dimensions: 21 × 17.5 mm
Datasheet
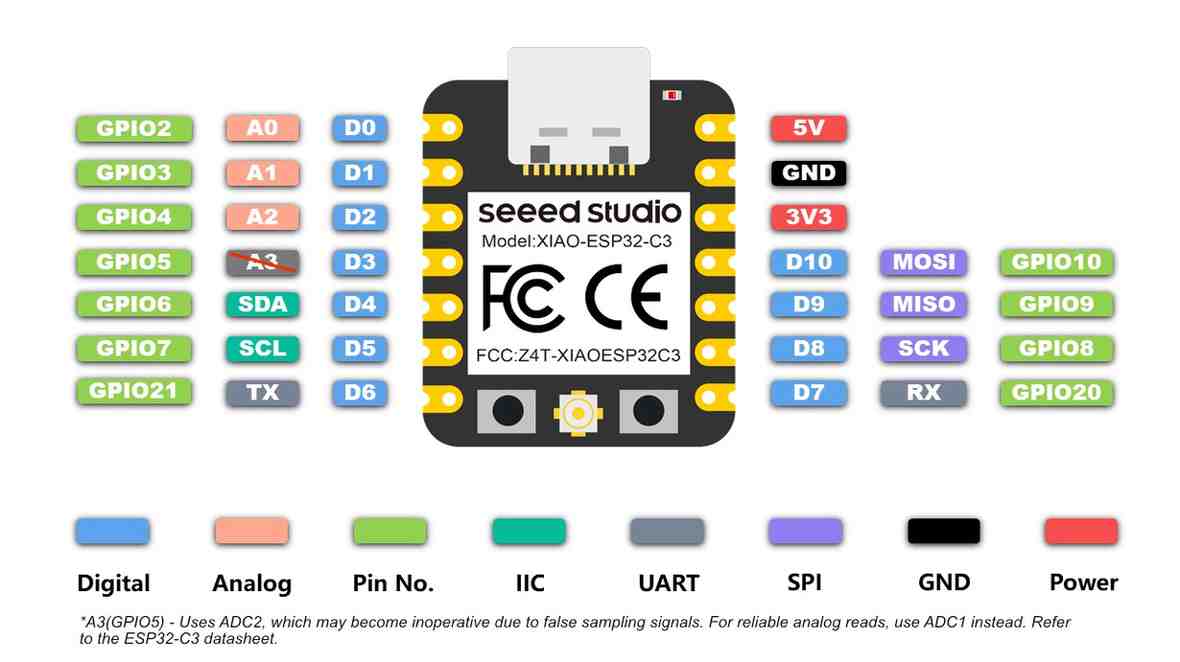
Wokwi
Wokwi is a web-based electronics simulator for Arduino, ESP32, Raspberry Pi, and AVR microcontrollers. It allows users to write, test, and debug embedded code in real time without physical hardware. The platform supports sensors, displays, motors, and communication modules. It is ideal for IoT, robotics, and embedded systems development.
Using this, I conducted two simulations with different boards: ESP32 and XIAO-ESP32-C3.
ESP32 with OLED display
I used an OLED display to obtain output with the ESP32, programming it in MicroPython.
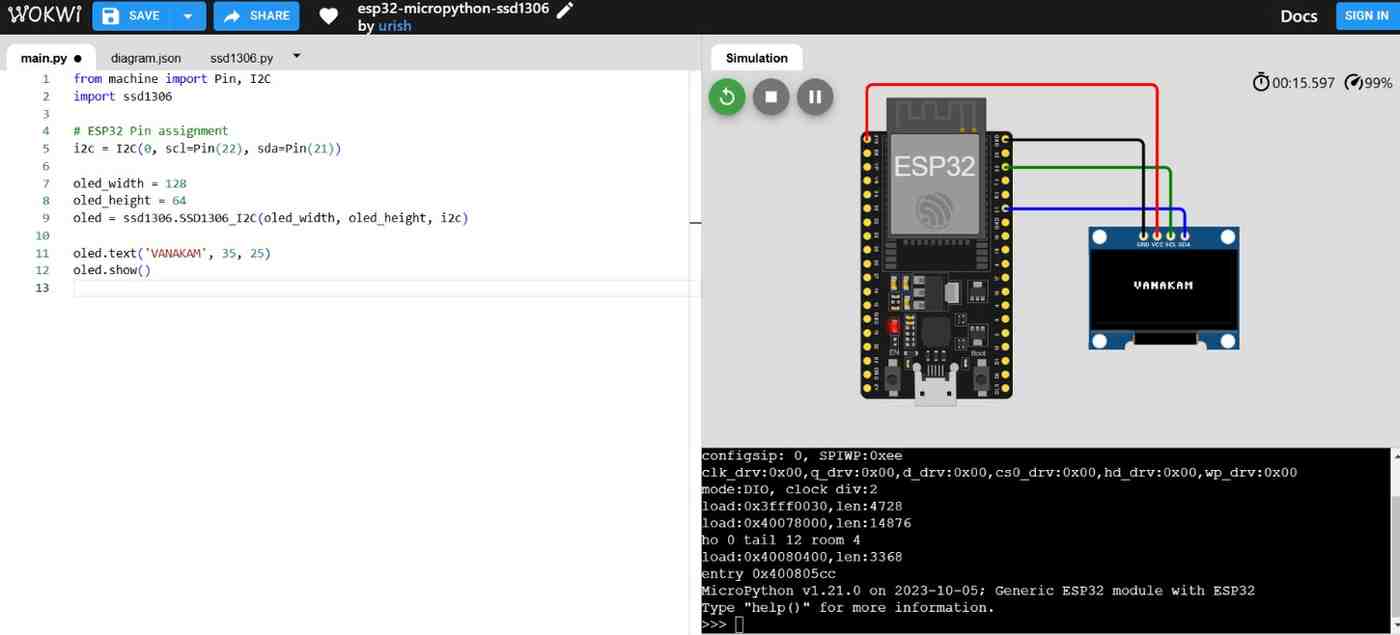
from machine import Pin, I2C
import ssd1306
# ESP32 Pin assignment
i2c = I2C(0, scl=Pin(22), sda=Pin(21))
oled_width = 128
oled_height = 64
oled = ssd1306.SSD1306_I2C(oled_width, oled_height, i2c)
oled.text('VANAKAM', 35, 25)
oled.show()
XIAO-ESP32-C3 with LED blink
I also experimented with LED blinking using this microcontroller.
void setup() {
Serial.begin(115200);
pinMode(D2, OUTPUT);
pinMode(D3, OUTPUT);
pinMode(D4, OUTPUT);
Serial.println("");
Serial.println("Let's Start");
}
void loop() {
Serial.println("Red");
digitalWrite(D2, HIGH);
delay(100);
digitalWrite(D2, LOW);
Serial.println("Yellow");
digitalWrite(D3, HIGH);
delay(100);
digitalWrite(D3, LOW);
Serial.println("Green");
digitalWrite(D4, HIGH);
delay(100);
digitalWrite(D4, LOW);
}